  |
|
 |
The goal of this assignment is to build and
program an autonomous robot that can locate a "fire" in a
burning "house" and put it out. | |
 |
|
 |
Assignment Details
From the Assignment
Webpage
The House and Fire:
The "house" will be a maze consisting of rigid
walls and obstacles. The layout of the house won't be known
beforehand but we can count on the following:
-
Except at wall junctions, there will be no
walls less than 24 inches apart.
-
The house will be enclosed (so that the robot
will not be able to wander off into the space beyond the
outer walls).
-
The walls and obstacles will always run
horizontally or vertically (in the coordinate system of the
outer walls). (Thus, they will always meet at right angles,
or as close as we can devise.)
-
The walls will be one foot high.
Here is an example of a bird's eye view of one
possible house (about 8' x 8'):
Some part of the house will be set on fire.
Rather, to simulate this, a lit candle will be placed on top
of a six-inch platform either against a wall, in a corner, or
between two walls in the house. Thus, the height of the flame
will be six inches plus whatever the height of the candle is.
(Leeway will be made to raise or lower the candle if it's the
"wrong" height for a particular mechanism a team has
constructed.) The robot will start in one of the four corners
of the house and will be aligned initially with the walls. The
goal of the robot will be to seek out the candle and
extinguish it. This is the highest-level goal -- other goals
include maintaining the robot's health and keeping it from
getting stuck.
Rules:
-
The robot should not push or ram any
wall/obstacle continuously, i.e., the intent is to keep the
house in its initial configuration. If a robot seems to be
pushing against a wall, it will be reset.
-
We can devise whatever mechanism we want to
extinguish the candle, as long as no water or fluids are
involved and no harm comes to the robot.
-
We may not use any glue or epoxy on our robot
(tape is OK) -- all equipment needs to be returned in its
original state.
Equipment:
The computational brains of the robots are
MIT's handyboards,
based on the 68HC11 microcontroller. Each team will have one
handyboard and a copy of Fred Martin's technical reference for
the handyboard. In addition, each team will have at least:
-
Three Lego motors
-
Two light sensors
-
One infrared sensor
-
Three touch sensors
There are spare sensors and possible spare
motors. We can use these as we see fit. Finally, there is a
pool of lego blocks with which to build the body of the
robot.
Interactive C:
The programming environment for the Handyboards
is Interactive C.
Here is an IC Online
Reference.
The Contest:
On Monday, February 5th each team will have to
demonstrate its robots' abilities. There will be at least
three trials for each robot on its own.
The first trial will involve a setup like the
one below. The robot is indicated by the green rectangle
(positioned upper left, facing toward the wall at the bottom
of the diagram), and the candle is represented by the yellow
circle (in the middle on the right).
The second trial will involve a similar set up,
in that the maze and robot starting position will be
identical, but the candle will be in an unknown location in
the maze.
The third trial will involve an unknown maze
(though not substantially different from the one above) and
unknown positions for the candle and robot.
Finally, if it seems feasible with the robot
designs that the teams have created, there will be a
head-to-head run with both robots located in an unknown (but
symmetric) environment at equal distance from the candle.
Judging Criteria:
In each case, the robots will be judged
according to these criteria:
-
Does the robot drive without getting stuck or
endangering itself?
-
Can the robot find the candle and indicate
that it knows that fact?
-
Can the robot extinguish the candle?
-
How quickly can it do
so? | |
 |
|
 |
Our Solution
Our main philosophy when desiging our robot
(affectionately known as Mr. Bizzaro) was the KISS mantra
(Keep It Simple, Stupid). In terms of the chassis, we aimed
for something small, agile, and relatively fast. Our initial
idea involved using a light sensor to detect the candle and a
snuffer to put the candle out (although our plans eventually
changed to using a fan instead of a snuffer). From the
beginning we knew that our program would be behavior based.
Initially we aimed for two behaviors: wander, which instructs
the robot to explore the maze until the candle is detected,
and avoid walls, which instructs the robot to reposition
itself if it runs into a wall. Our final program ended up
using four behaviors, but it operates using the same basic
idea as our original software concept.
| |
 |
|
 |
The Chassis
The chassis started out as a simple
three-wheeled, short, squat, frame. The wheels were arranged
in a triangle shape, with the two larger back wheels connected
to Lego Mindstorm
motors and the front wheel left alone as an independent
castor. Next a container was built to hold the handyboard
vertically on top of the frame. Two bump sensors were added to
the "front," and a light sensor tube was added to the top of
the container. At this point the robot became a little top
heavy and unbalanced, so a fourth castor was added in the
back, making the frame of wheels diamond-shaped. The only
piece of hardware left to add was the snuffer. We were a
little perplexed about how to get the snuffer to extend and
retract until in a brilliant stroke of genius Aaron
constructed a crank-piston system entirely out of legos. After
testing our robot several times, we realized that getting our
robot to stop at the correct snuffing distance away from the
candle would be extremely difficult. We also realized that a
fan would be much more practical as it could be turned on as
soon as light was detected and we wouldn't have to worry about
stopping at all. After this insight we decided to essentially
re-build the entire chassis. Not only did we solve the problem
of how to easily extinguish the candle, but we were also able
to go back and fix some of the design flaws that were bugging
us (high center of gravity, too narrow base, off balance
wheels, stiff castors, etc.)
The base frame of the new chassis is very
similar to the original frame of the old chassis. There are
three wheels in a triangular formation, two large ones in the
front and a trackball mounted in a cylinder in the back. The
handyboard is mounted in the back, on edge, with all of the
ports facing out. The two bump sensors are mounted at the
front. The light tube sits on top of a platform that is
exactly six inches above the ground (the height of the
candle). The fan is attached to the top of the light tube and
is angled downwards. This allows the air generated by the fan
(which is focused through a rectangular tube) be aimed
directly at the flame.
Here are some pictures of Mr. Bizzaro:
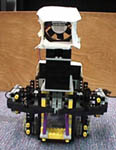 |
This is the "front" of the robot. You can
see the two bump sensors in the front, as well as the
two larger wheels (they're gray and narrow). The fan and
rectangular focusing tube are sitting on top of the
light tube. You can click on all of these pictures (as
well as the pic of the prototype above) to enlarge
them. |
This is the back of the robot. The white
square at the bottom of the picture is the trackball
housing. Above that, you can see the handyboard. The
back of the fan is at the very top. |
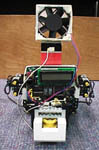 |
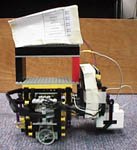 |
This is the left side of the robot. You
can see the gray wheel at the very bottom. The long,
black, rectangular thing at the top is the side of the
light tube. The thing covered in masking tape is the
left edge of the handyboard, and the white square is the
trackball housing. |
This is the right side of the robot.
Again you can see the wheel at the bottom, the light
tube and focusing tube at the top, and the edges of the
handyboard and trackball housing at the back. |
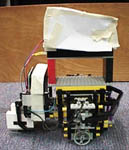 |
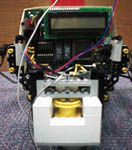 |
This is a closeup of the back. You can
see the trackball housing and handyboard. |
This is a closeup of the left
wheel. |
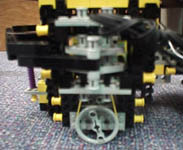 |
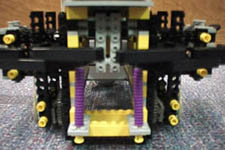 |
This is a closeup of the front. You can
mainly see the two bump sensors edge on, as well as the
two gray
wheels. | | |
 |
|
 |
The Program
Our program uses basic subsumption architecture
to regulate the robot's actions. We have four main behaviors:
-
Avoid Walls
-
Find Light
-
Wander Spin
-
Wander Drive
The behavior with the lowest priority is wander
drive. The robot executes the wander drive behavior by driving
forward for a set amount of time (right now, that set amount
of time is set to five seconds). In wander drive the left
motor is set to spin slightly faster than the right. This
effectively gives the robot a drift to the right. Eventually
will hit the right wall of the maze, back up, turn slightly,
and travel forward (again with a drift to the right). This
results in the robot effectively following the right wall
(perhaps we should have called this behavior follow wall?).
The next behavior in the hierarchy is wander spin. After the
set amount of time in the wander drive behavior has passed,
the robot will stop and turn in a full 360 degree circle. If
the light sensor detects light, the robot will enter the next
highest behavior, find light. When this behavior is entered
the fan is turned on and the robot moves in the direction that
the light was detected. If the sensor doesn't detect light in
wander spin, the robot will go back into the wander drive
behavior. The behavior with the highest priority is avoid
walls. If at any time one of the robot's bump sensors is
tripped, the robot will immediately stop, back up, turn (the
direction of the turn depends on which bump sensor was
tripped), and then re-enter whichever behavior it was
previously in.
You can find a copy of our code here. | |
 |
|
 |
Problems We
Encountered
By far the biggest problem we encountered was
our battery running out after ~20 minutes of use. This ended
up preventing us from fully testing our robot. Other than
that, most of the problems we encountered had to do with
various aspects of the chassis. A lot of these problems were
fixed when we re-designed the chassis.
The first problem we encountered was the
coefficient of friction on our castor being too high.
Everytime our robot switched from turning in a circle to
moving towards the light, it would quickly get off track. This
was caused by the castor remaining in a turned position and
not straightening out when the robot began to move forwards.
We fixed this problem by making the back wheel a trackball.
Now, whenever the robot moves out of a turn and towards the
light it moves in a straight path.
Another problem we had was with the robot's
center of gravity being way too high. This was caused by us
adding hardware to the robot in a solely vertical direction.
We fixed this by re-building the chassis and making the frame
of the robot wider and squatter.
A relatively major problem we had was that the
fan was too weak. Even with the fan physically touching the
candle, the force of the air was not enough to blow the candle
out. We fixed this problem by creating a rectangular focusing
tube. Now the fan will extinguish the candle approximately 80%
of the time.
We discovered a fairly serious problem this
evening (2/5/01) when our robot tried to navigate a corner of
the maze. First it would run into one wall of the corner
setting off the left bump sensor. The robot would then back
up, turn right, and move forward, hitting the other wall and
setting off its right bump sensor. Then the robot would back
up, this time turn left, move forward, and hit the first wall
again, setting off the right bump sensor. The robot ended up
effectively trapped in the corner. We solved this problem by
making the robot turn more (now ~90 degrees instead of the
previous ~45) after it backs up following a
collision. | |
 |
|
 |
Evaluation
Because the battery kept running out before we
could thoroughly test our robot, we are not certain that he
can naviagate the maze and accomplish his goal of
extinguishing the candle 100% of the time. Most of the
problems with the robot's performance have to do with the fan.
Preliminary tests indicate that once the light is detected,
the robot can put out the candle (with the use of the fan)
about 80% of the time. The other 20% of the time the robot
ends up running into the candle and knocking it over before
the fan can put out the flame. The air resistance flowing
around the candle as it falls from the platform is enough to
extinguish the flame, and while this technically accomplishes
our goal, we do not feel it does so in the safest manner
possible.
In our tests the robot detected the light about
50% of the time. This, however, was using an old wander
behavior. The battery ran out tonight (2/5/01) before we could
fully test our new "follow wall" wander behavior. The only
problem with the old behavior was that it was so random that
we didn't have the patience to sit around and see if the robot
would get lucky enough to wander near the light. We do feel,
however, that given an infinite amount of time, the robot
could have found the light using the old wander behavior. As
soon as we have tested our new follow wall behavior, I will
post an updated evaluation here.
Mr. Bizzaro attempted to demonstrate his
amazing extinguishing abilities this afternoon (2/6/01), but
he seemed to suffer from a bit of stage fright. After testing
out our new "follow wall" code we noticed that the drift was
not pronounced at all, and that the robot was spinning more
than 360 (and thereby getting off track) when searching for
light. After making the drift much more pronounced and
reducing the time the robot spent spinning, we set him at his
task again. We noticed pretty much immediately that there was
a significant drawback to the drift. Because the robot was
constantly bumping into the wall, it never achieved the full 5
seconds of uninterrupted driving, and therefore didn't go into
many spins searching for light. We placed the robot close to
the candle to see if it would detect the light and put it out
(which it has done accurately many times before). It
recognized the light, turned on the fan, and drove towards the
candle, but due to the fact the other team had slightly
lowered the platform (grr!), the fan was not at the correct
angle and wasn't able to extinguish the flame (of course, the
fact that the fan motor was really wussy could also have
affected its extinguishing abilities...) We also noticed that
if the robot didn't immediately extinguish the candle, it
would run into it and enter the avoid walls behavior, which
made it back up, turn, and lose the candle from its line of
sight. It would have been nice if the robot could distinguish
between running into the candle and running into a wall, but
I'm not sure how we could've implemented that accurately. In
retrospect, using IR sensors to follow the wall would've been
much more effective. Also it seems that our random wander
behavior which relied on the robot getting lucky was more
effective than our follow wall behavior.
| |
 |
|
 |
Mr. Bizzaro in Action
Mr. Bizzaro will probably be demonstrating his
abilities and competing against the other team sometime before
this thursday (2/8/01). At the demonstration I'll take some
digital movies of Mr. Bizzaro in action and post them
here. | |
 |
|
 |
|
 | |