- Project Objectives
- First-Half Progress
Our modified goals for the project midterm are as follows:
- Build a motorized trash collector and attach it to our existing robot
- Update our robot to incorporate an additional bump sensor
- Test out the sonar sensor and ensure that it is functioning properly
- Research evidence grids to determine how we will use them with our robot
We divided the work between the three of us so that we were working on separate
parts independently. Ryan worked on the bump sensors and the overall robot
architecture, Conor built the trash collector, and Paul tested the sonar sensor
and looked at evidence grid information. Once we were done we combined our work for
a temporary unfinalized working robot.
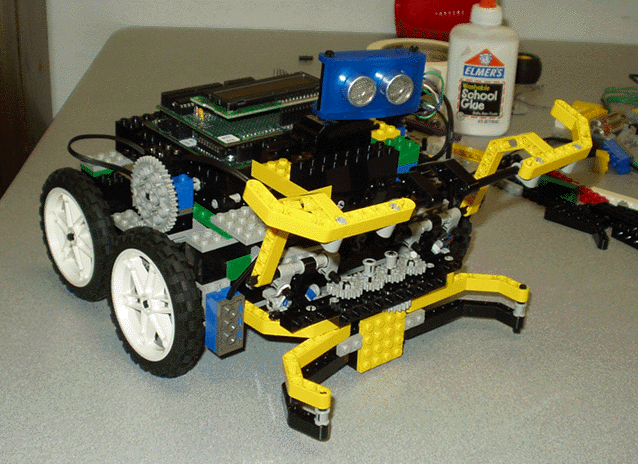
Figure 1 - Table Sweeper v.3.0
Our new bump sensors are on both front corners, and are more stable than the one in
our previous design. This is partially due to them needing to cover less area than
our previous one, and partially due to our experience working with the first one.
The legos are much closer to the actual sensors now, increasing their sensitivity
and reliability.
With the trash collector, we focused on simply getting one built, and not yet
worrying about its control. To get ideas on how to accomplish it, we built a
sample robotic arm described in a Lego book in the lab. Once we had the completed
arm, we scaled it down and fit it for our robot. The angled collector pieces look
almost the same as the ones in the previous, stationary design, but in the new
design there are a number of gears which permit the motion of the collector arms.
We attached a 40-tooth gear to the rotating part of a motor, and another 40-tooth
gear to the first 40-tooth gear. This 40-tooth gear was attached, via a rod, to a
16-tooth gear. This 16-tooth gear was attached in series with 3 other 16-tooth gears
which had rods running through them. Two of these rods had collector arms attached
to them, which rotate in unison to create our desired effect. It was pretty tough
arranging and rearranging the number of tiny legos needed to create this part, but
the final component should work pretty well once we write the code for it.
With these two new major components completed, the balance of the system had shifted
such that our robot was more front-heavy than it was before the modifications. We
shifted the weight of the system and added some wheels to stabilize it. We also
moved forward the wheels we already had in place, and replaced some of the hacked
together pieces we had before now that the Joe Malone problem was a thing of the
past. We also put on the wheel encoders and the light sensors in preparation of
using evidence grids. Again, the goal here was to get the hardware of the robot
as ready as possible for the next round of coding we will do.
This evidence grid programming is what Paul looked into. While we are still not
totally sure about how we are going to do this, we have some general ideas. We are
going to be using a fixed sensor model. We will build a map with sensor readings
using evidence grids. Once we have a sufficiently good map (still to be determined
how we will determine this threshold), we will clear the table. Since we have a
motorized trash collector now, our table clearing should be improved over the first
system. We do not plan on detecting the trash, but rather clasping the trash
collector whenever the robot turns so that we will hopefully gather all of the
trash the robot has at the time in order to be pushed off the table.
The other hardware component we got working was the sonar sensor. We successfully
got it talking with our Handy Board using some code that another group had written
up. Their program plays a tone that varies depending on how far the object is from
the sonar sensor. We are convinced that with the proper hand movements, we could
reproduce the infamous "Hamster Dance" theme. These are the kinds of things one
comes up with after tinkering with legos for four straight hours.
Finally, here are a couple pseudo-code files we have, a first step towards
implementing our final system.
Table Sweeper
Sonar Code
- Second-half Progress
With our much-improved robot, we set out to devise an algorithm for table clearing.
We wanted to break the table into a series of grid cells. Each cell would have a
probability for containing an obstacle. These probabilities would be initially the
same for all cells (like 0.5). Our robot would start in cell (0,0) and use the
sonar to scan the table. It then would update the table of probabilities depending
on how far away the obstacles were. Once the cells adjacent to the robot were
updated, the robot would have to move. It would look to its left, center, and right
for a possible next step, ignoring directions that were edges of the table. It would
travel in the direction of the cell with the lowest probability of having an obstacle.
If all possibilities were above our threshold then they all likely have obstacles in
them. In this case, the robot would turn around and go in the direction from whence
it came. We thought that if this approach worked then the bump sensors and light
sensors would be redundant, since the sonar and encoders should do all the work.
Here is initial code we developed to control our robot:
thefranchise.ic.
While this algorithm may have worked for the Nomad simulator, the real world is not
so kind. We ran into two major hurdles regarding our assumptions. Our main
assumption was that we would be able to perfectly keep track of the robot's
movements via the encoders. This turned out to be a bad assumption. Our encoders
did not compute the same values. One of the encoders recorded 16 clicks per
rotation while the other recorded only 8 clicks per rotation. We tried to compensate
for this but we still could not get the encoders to work the way we wanted. Another
problem was that we could not get the wheels to move perfectly straight. This would
present problems when trying to map out the robot's movement according to grid cells.
After about five hours of trying to get the encoders/wheels to work so that we could
get evidence grid mapping to work we abandoned the idea, because we would never get
them to work as precisely as needed for our design to succeed. Instead we tried to
make the best reactive-controlled robot that we could. This robot would always
avoid walls using the sonar and clasp the gripper whenever it turned, both improvements
upon our first tablesweeper. We started by taking our code from the first tablesweeper
and adding the changes necessary to reflect our new design. We had added an additional
bump sensor, an additional light sensor, and the sonar, but since the bump sensor and
light sensor were just redundant additions and we already had code for the sonar we
thought these changes would not take very long.
The code we started with would not compile initially, and when we debugged it we found
some glaring omissions that should have prevented the first robot from working such as
left-out semicolons. At this point, the network was down, so we could not verify that
we had the same code, so we made do with what we had. Eventually we got the code to
work and we made our additions, but we still had some problems with the physical robot.
The sonar sat too low, so that when it rotated on the servo the sonar would detect the
jungle of wires taped together. We corrected this problem by raising the sonar.
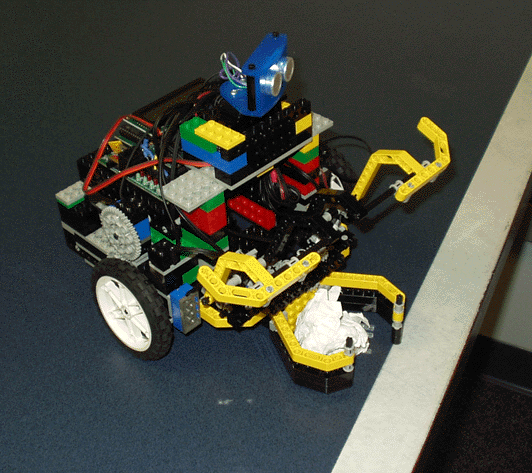
Figure 2 - Table Sweeper v.3.1
The random additions we kept making to the chassis of the robot had a cumulative
effect on its stability. The added weight on the chassis applied more torque on the
wheels. Since the wheels were pretty large, the axles bent down, forcing the wheels to
point slightly inward at the top. This caused them to touch the side of the chassis
slightly, which then caused the wheels to move unpredictably. We were never able to
adequately fix this problem.
We spent the rest of our time trying to make an adequate system with what we had working.
Eventually we got a working sonar, wheels that moved somewhat predictably, working
light sensors (one lego and one non-lego), but the bump sensors started failing. Ryan
swears to think instant that the bump sensor problem is a code one, not a mechanics
problem (editor/Conor's note: the gripper, my creation, worked perfectly throughout).
Here is the final reactive code we utilized to control our robot:
tablesweep.ic.
- Perspective
If we were to start over, what would we do differently? Make the chassis more narrow,
lower the robot's center of gravity, and use smaller wheels. This would hopefully fix
the motion problems we had. Also, we would test out the encoders separately before
ensconsing them in our system so that we would start with two encoders that performed
identically. Finally, we would start with a simpler code base instead of designing a
grand algorithm that may or may not be practical depending upon the limitations of the
system. If there is anything we have learned in Robotics, both from the Rugwarrior and
the Handyboard/lego robot, it is that the best code in the world does not mean a thing
if the physical system you are working with does not perform as you expect.